Automating Proxmox Snapshots with a Custom Bash Script
Automate Proxmox snapshots with a bash script
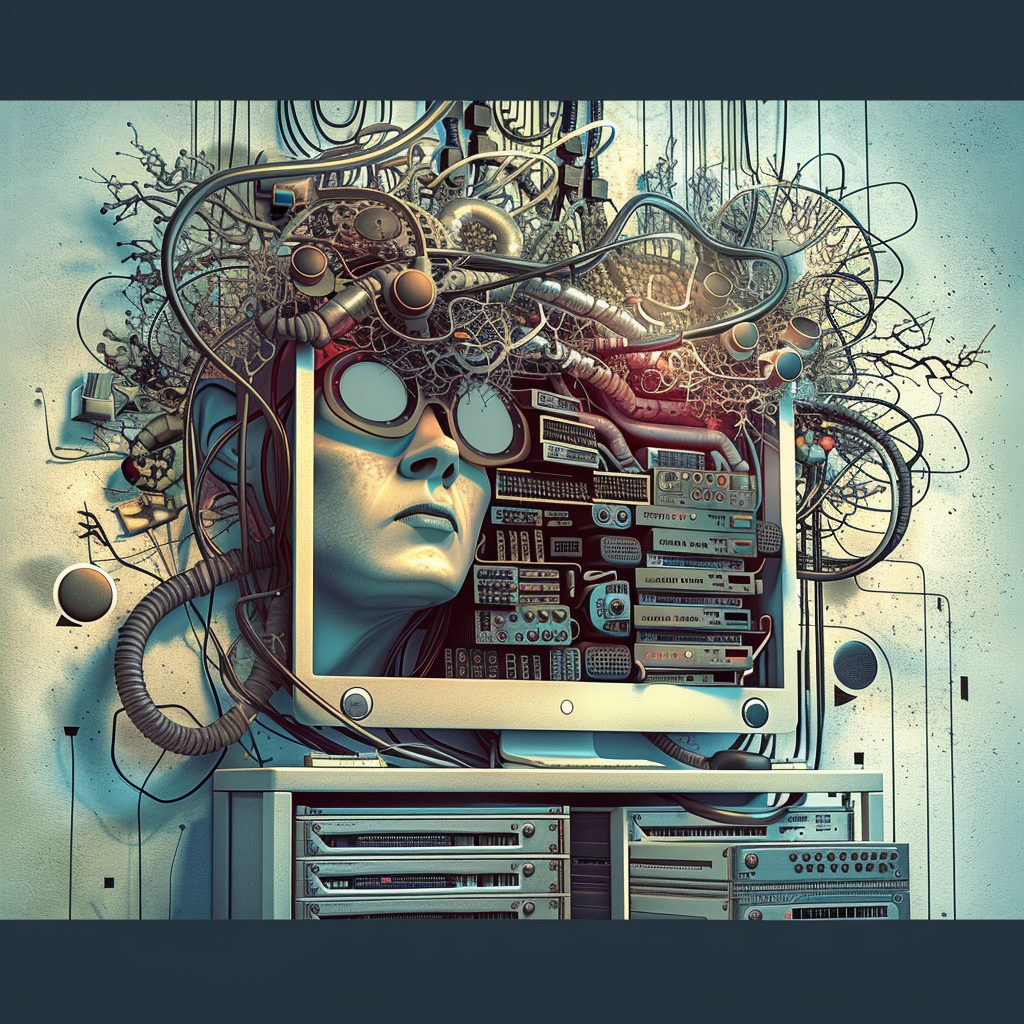
Automating VM Snapshots with a Custom Bash Script
When managing a cluster of virtual machines (VMs), ensuring that you have regular snapshots is critical for maintaining data integrity and recovery options. Automating this process can save a significant amount of time and reduce the risk of human error. In this blog post, we'll explore a bash script that automates the snapshot process for VMs within a specified range of IDs.
Snapshots are a vital part of VM management, allowing you to capture the state of a machine at a specific point in time. This can be invaluable for disaster recovery, software testing, and more. However, manually creating snapshots for each VM can be tedious and error-prone, especially in larger environments.
To streamline this process, we'll create a bash script that:
- Defines the range of VM IDs to target.
- Uses an associative array to map node names to their IP addresses.
- Retrieves the list of VMs in the cluster.
- Takes snapshots of VMs within the specified ID range.
Let's dive into the script and see how it works.
The Script
Here’s the complete script for automating VM snapshots:
#!/bin/bash
# Define the range of VM IDs
start_vmid=5000
end_vmid=6999
# Define a bash associative array with node names as keys and their corresponding IPs as values
declare -A node_ips=(
["pve_node_name"]="pve_node_ip"
["pve_node2_name"]="pve_node2_ip"
)
# Snapshot name
snapshot_name="snapshot_$(date +%Y%m%d_%H%M%S)"
# Get list of all VM IDs in the cluster
vm_ids=$(pvesh get /cluster/resources --type vm --output=json | jq -r '.[] | select(.type=="qemu") | "\(.node)/\(.vmid)"')
# Take snapshot of each VM within the specified ID range
for vm in $vm_ids; do
node_name=$(echo $vm | cut -d'/' -f1)
vmid=$(echo $vm | cut -d'/' -f2)
# Lookup IP address using the node name from the mapping
node_ip="${node_ips[$node_name]}"
if [[ $vmid -ge $start_vmid && $vmid -le $end_vmid ]]; then
echo "Taking snapshot of VM $vmid on node $node_name ($node_ip) with snapshot name $snapshot_name"
ssh root@$node_ip "qm snapshot $vmid $snapshot_name" &
else
echo "Skipping VM $vmid - outside target range."
fi
done
wait
echo "Snapshots taken for target VMs."
Breakdown of the Script
- Define VM ID Range:
At the beginning of the script, we define the range of VM IDs that we want to target for snapshots. This is done using two variables:start_vmid
andend_vmid
. Adjust these variables according to your needs. - Node IP Mapping:
We use a bash associative array to map node names to their respective IP addresses. This allows the script to identify and connect to the correct node for each VM. - Generate Snapshot Name:
The snapshot name is dynamically generated using the current date and time, ensuring each snapshot has a unique identifier. - Retrieve VM List:
The script usespvesh
andjq
to retrieve a list of all VMs in the cluster, filtering only for those of type "qemu". This list includes both the node name and VM ID. - Snapshot Process:
The script loops through the list of VMs, checks if the VM ID falls within the specified range, and then initiates a snapshot via SSH for those that do. VMs outside the target range are skipped. - Wait for Completion:
Thewait
command ensures that all background snapshot processes complete before the script finishes.
Feel free to customize the script to fit your specific needs, such as changing the VM ID range, modifying the node IP mappings, or adjusting the snapshot naming convention. With this automation in place, you'll spend less time on routine maintenance tasks and more time focusing on what really matters.